curl-library
Sending an e-mail
Date: Tue, 21 Dec 2010 23:32:30 +0200
I am trying to send an email through SMTP and gmail. I get output error
'Login denied' (see attached screenshot for output). I am sure that the
login and the password are correct. I triple-tested them! I am trying to
make a simple program in C++ in order to automate a bit the mailing process
(there are good tools for this but I found them "heavy" for any system!), so
I want to make something "light" for my own purposes only! Note that I am
trying to attach a file to this email (my_attachment.tar.gz)
This is the code I use:
*#include <stdio.h>
#include <string.h>
#include <curl/curl.h>
#include <stdlib.h>
#define FROM "mymail_at_gmail.com"
#define TO "mymail_at_gmail.com"
static const char *payload_text[]={
"Date: Mon, 29 Nov 2010 21:54:29 +1100\n",
"To: " TO "\n",
"From: " FROM "(Example User)\n",
"Message-ID: <dcd7cb36-11db-487a-9f3a-e652a9458efd_at_rfcpedant.example.org
>",
"Subject: Hello!\n",
"\n",
"See attachments ;).\n",
"\n",
"Still reading?\n",
"Check RFC5322.\n",
NULL
};
struct upload_status {
int lines_read;
};
static size_t payload_source(void *ptr, size_t size, size_t nmemb, void
*userp)
{
struct upload_status *upload_ctx = (struct upload_status *)userp;
const char *data;
if ((size == 0) || (nmemb == 0) || ((size*nmemb) < 1)) {
return 0;
}
data = payload_text[upload_ctx->lines_read];
if (data) {
size_t len = strlen(data);
memcpy(ptr, data, len);
upload_ctx->lines_read ++;
return len;
}
return 0;
}
int main(void)
{
CURL *curl;
CURLcode res;
struct curl_slist *recipients = NULL;
struct upload_status upload_ctx;
upload_ctx.lines_read = 0;
curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, "smtp://smtp.gmail.com:587");
curl_easy_setopt(curl, CURLOPT_USE_SSL, CURLUSESSL_ALL);
curl_easy_setopt(curl, CURLOPT_FILE, "my_attachment.tar.gz");
curl_easy_setopt(curl, CURLOPT_USERNAME, "mymail_at_gmail.com");
curl_easy_setopt(curl, CURLOPT_PASSWORD, "mypaswd");
curl_easy_setopt(curl, CURLOPT_MAIL_FROM, FROM);
recipients = curl_slist_append(recipients, TO);
curl_easy_setopt(curl, CURLOPT_MAIL_RCPT, recipients);
curl_easy_setopt(curl, CURLOPT_READFUNCTION, payload_source);
curl_easy_setopt(curl, CURLOPT_READDATA, &upload_ctx);
curl_easy_setopt(curl, CURLOPT_VERBOSE, 1);
res = curl_easy_perform(curl);
curl_slist_free_all(recipients);
curl_easy_cleanup(curl);
}
return 0;
}
*Thx in advance!
-------------------------------------------------------------------
List admin: http://cool.haxx.se/list/listinfo/curl-library
Etiquette: http://curl.haxx.se/mail/etiquette.html
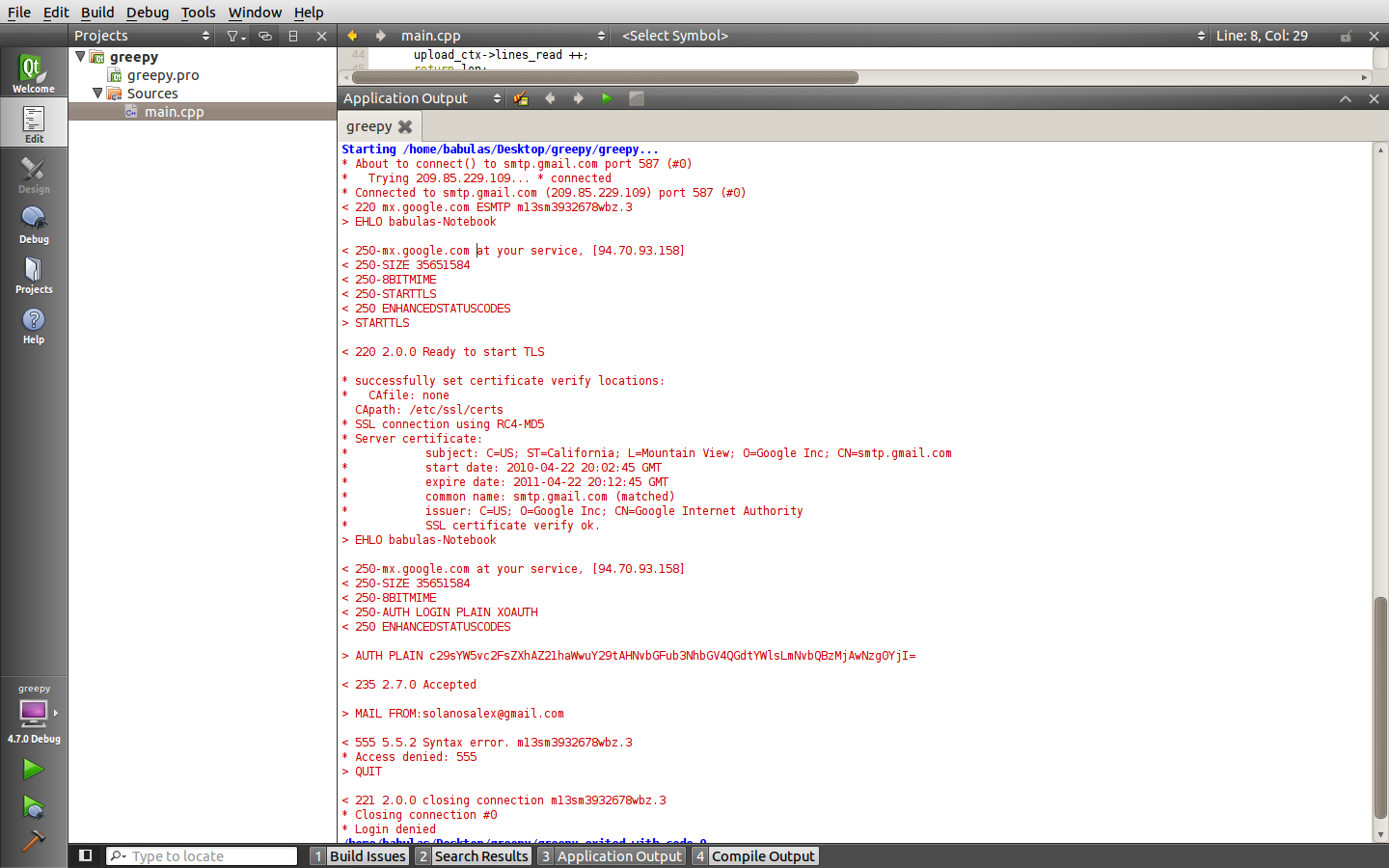